Learn how to perform ETL (Extract, Transform, Load) processes using PySpark. This comprehensive guide covers everything from environment setup and data extraction to advanced data transformations, handling missing values, data type conversion, and performance tuning.
You’ll also explore advanced topics like stream processing, machine learning integration, and working with large text data. Whether you’re a beginner or an experienced user, this guide has something for everyone looking to master ETL processes with PySpark.
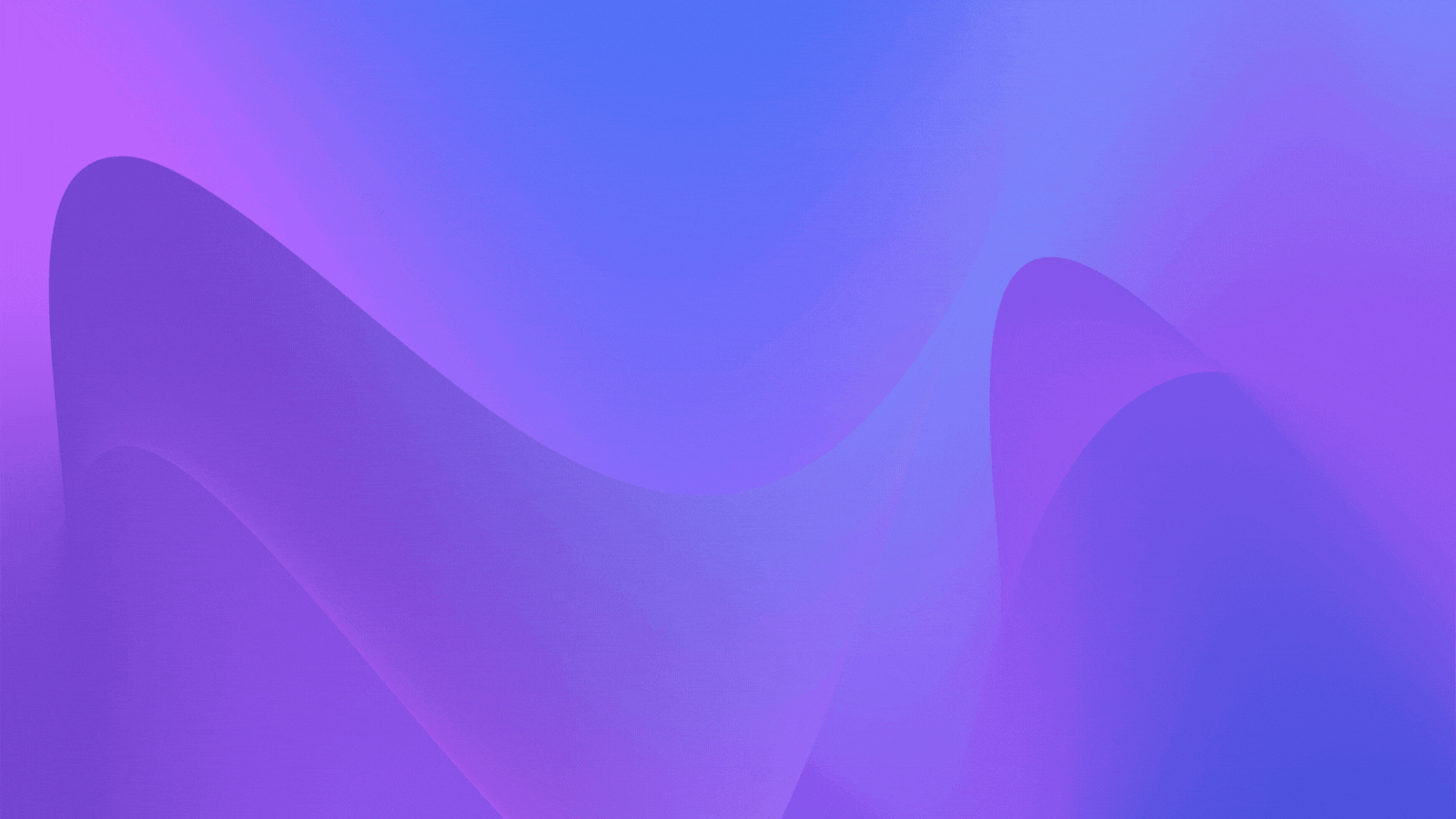
Quick Summary
Environment Setup and SparkSession Creation
- Install PySpark:
pip install pyspark
- Start a SparkSession:
from pyspark.sql import SparkSession spark = SparkSession.builder.appName('ETL Process').getOrCreate()
- Install PySpark:
Data Extraction
- 📊 Read Data from CSV:
df = spark.read.csv('path/to/csv', inferSchema=True, header=True)
- 📋 Read Data from JSON:
df = spark.read.json('path/to/json')
- 📁 Read Data from Parquet:
df = spark.read.parquet('path/to/parquet')
- 🗃️ Read Data from a Database:
df = spark.read.format("jdbc") \ .option("url", jdbc_url) \ .option("dbtable", "table_name") \ .option("user", "username") \ .option("password", "password") \ .load()
- 📊 Read Data from CSV:
Data Transformation
- 🔄 Selecting Columns:
df.select('column1', 'column2')
- 🔍 Filtering Data:
df.filter(df['column'] > value)
- ➕ Adding New Columns:
df.withColumn('new_column', df['column'] + 10)
- 🔄 Renaming Columns:
df.withColumnRenamed('old_name', 'new_name')
- 📊 Grouping and Aggregating Data:
df.groupBy('column').agg({'column2': 'sum'})
- 🔗 Joining DataFrames:
df1.join(df2, df1['id'] == df2['id'])
- 🔍 Sorting Data:
df.orderBy(df['column'].desc())
- 🗑️ Removing Duplicates:
df.dropDuplicates()
- 🔄 Selecting Columns:
Handling Missing Values
- 🚫 Dropping Rows with Missing Values:
df.na.drop()
- 🔄 Filling Missing Values:
df.na.fill(value)
- 🔄 Replacing Values:
df.na.replace(['old_value'], ['new_value'])
- 🚫 Dropping Rows with Missing Values:
Data Type Conversion
- 🔢 Changing Column Types:
df.withColumn('column', df['column'].cast('new_type'))
- 📅 Parsing Dates:
from pyspark.sql.functions import to_date df.withColumn('date', to_date(df['date_string']))
- 🔢 Changing Column Types:
Advanced Data Manipulations
- 💼 Using SQL Queries:
df.createOrReplaceTempView('table') spark.sql('SELECT * FROM table WHERE column > value')
- 🪟 Window Functions:
from pyspark.sql.window import Window from pyspark.sql.functions import row_number df.withColumn('row', row_number().over(Window.partitionBy('column').orderBy('other_column')))
- 🔄 Pivot Tables:
df.groupBy('column').pivot('pivot_column').agg({'column2': 'sum'})
- 💼 Using SQL Queries:
Data Loading
- 💾 Writing to CSV:
df.write.csv('path/to/output')
- 💾 Writing to JSON:
df.write.json('path/to/output')
- 💾 Writing to Parquet:
df.write.parquet('path/to/output')
- 💾 Writing to a Database:
df.write.format("jdbc") \ .option("url", jdbc_url) \ .option("dbtable", "table_name") \ .option("user", "username") \ .option("password", "password") \ .save()
- 💾 Writing to CSV:
Performance Tuning
- 🚀 Caching Data:
df.cache()
- 📡 Broadcasting a DataFrame for Join Optimization:
from pyspark.sql.functions import broadcast df1.join(broadcast(df2), df1['id'] == df2['id'])
- 🔄 Repartitioning Data:
df.repartition(10)
- 📦 Coalescing Partitions:
df.coalesce(1)
- 🚀 Caching Data:
Debugging and Error Handling
- 🐞 Showing Execution Plan:
df.explain()
- 🛠️ Catching Exceptions during Read: Implement try-except blocks during data reading operations.
- 🐞 Showing Execution Plan:
Working with Complex Data Types
- 💥 Exploding Arrays:
from pyspark.sql.functions import explode df.select(explode(df['array_column']))
- 📊 Handling Struct Fields:
df.select('struct_column.field1', 'struct_column.field2')
- 💥 Exploding Arrays:
Custom Transformations with UDFs
- 🛠️ Defining a UDF:
from pyspark.sql.functions import udf @udf('return_type') def my_udf(column): return transformation
- 🔄 Applying UDF on DataFrame:
df.withColumn('new_column', my_udf(df['column']))
- 🛠️ Defining a UDF:
Working with Large Text Data
- 📝 Tokenizing Text Data:
from pyspark.ml.feature import Tokenizer Tokenizer(inputCol='text_column', outputCol='words').transform(df)
- 📊 TF-IDF on Text Data:
from pyspark.ml.feature import HashingTF, IDF HashingTF(inputCol='words', outputCol='rawFeatures').transform(df)
- 📝 Tokenizing Text Data:
Machine Learning Integration
- 🤖 Using MLlib for Predictive Modeling: Building and training machine learning models using PySpark’s MLlib.
- 🎯 Model Evaluation and Tuning:
from pyspark.ml.evaluation import MulticlassClassificationEvaluator MulticlassClassificationEvaluator().evaluate(predictions)
Stream Processing
- 🌊 Reading from a Stream:
dfStream = spark.readStream.format('source').load()
- 💾 Writing to a Stream:
dfStream.writeStream.format('console').start()
- 🌊 Reading from a Stream:
Advanced Data Extraction
- 📊 Reading from Multiple Sources:
df = spark.read.format('format').option('option', 'value').load(['path1', 'path2'])
- 🔄 Incremental Data Loading: Implementing logic to load data incrementally, based on timestamps or log tables.
- 📊 Reading from Multiple Sources:
Complex Data Transformations
- 🔄 Nested JSON Parsing:
from pyspark.sql.functions import json_tuple df.select(json_tuple('json_column', 'field1', 'field2'))
- 🗺️ Applying Map-Type Transformations: Using map functions to transform key-value pair data.
- 🔄 Nested JSON Parsing:
Advanced Joins and Set Operations
- 🚀 Broadcast Join with Large and Small DataFrames: Utilizing broadcast for
efficient joins.
– 🔄 Set Operations (Union, Intersect, Except):
df1.union(df2), df1.intersect(df2), df1.except(df2)
Data Aggregation and Summarization
- 📊 Complex Aggregations:
df.groupBy('group_col').agg({'num_col1': 'sum', 'num_col2': 'avg'})
- 📊 Rollup and Cube for Multi-Dimensional Aggregation:
df.rollup('col1', 'col2').sum(), df.cube('col1', 'col2').mean()
- 📊 Complex Aggregations:
Advanced Data Filtering
- 🔍 Filtering with Complex Conditions:
df.filter((df['col1'] > value) & (df['col2'] < other_value))
- 🔍 Using Column Expressions:
from pyspark.sql import functions as F df.filter(F.col('col1').like('%pattern%'))
- 🔍 Filtering with Complex Conditions:
Working with Dates and Times
- 📅 Date Arithmetic:
df.withColumn('new_date', F.col('date_col') + F.expr('interval 1 day'))
- 🕒 Date Truncation and Formatting:
df.withColumn('month', F.trunc('month', 'date_col'))
- 📅 Date Arithmetic:
Handling Nested and Complex Structures
- 🔄 Working with Arrays and Maps:
df.select(F.explode('array_col')), df.select(F.col('map_col')['key'])
- 📋 Flattening Nested Structures:
df.selectExpr('struct_col.*')
- 🔄 Working with Arrays and Maps:
Text Processing and Natural Language Processing
- 📜 Regular Expressions for Text Data:
df.withColumn('extracted', F.regexp_extract('text_col', '(pattern)', 1))
- 😊 Sentiment Analysis on Text Data: Using NLP libraries to perform sentiment analysis on textual columns.
- 📜 Regular Expressions for Text Data:
Advanced Window Functions
- 🪟 Window Functions for Running Totals and Moving Averages:
from pyspark.sql.window import Window windowSpec = Window.partitionBy('group_col').orderBy('date_col') df.withColumn('cumulative_sum', F.sum('num_col').over(windowSpec))
- 🥇 Ranking and Row Numbering:
df.withColumn('rank', F.rank().over(windowSpec))
- 🪟 Window Functions for Running Totals and Moving Averages:
Data Quality and Consistency Checks
- 📊 Data Profiling for Quality Assessment: Generating statistics for each column to assess data quality.
- 🔍 Consistency Checks Across DataFrames: Comparing schema and row counts between DataFrames for consistency.
ETL Pipeline Monitoring and Logging
- 📝 Implementing Logging in PySpark Jobs: Using Python’s logging module to log ETL process steps.
- 📈 Monitoring Performance Metrics: Tracking execution time and resource utilization of ETL jobs.
ETL Workflow Scheduling and Automation
- ⏰ Integration with Workflow Management Tools: Automating PySpark ETL scripts using tools like Apache Airflow or Luigi.
- 🔄 Scheduling Periodic ETL Jobs: Setting up cron jobs or using scheduler services for regular ETL tasks.
Data Partitioning and Bucketing
- 🗃️ Partitioning Data for Efficient Storage:
df.write.partitionBy('date_col').parquet('path/to/output')
- 🪣 Bucketing Data for Optimized Query Performance:
df.write.bucketBy(42, 'key_col').sortBy('sort_col').saveAsTable('bucketed_table')
- 🗃️ Partitioning Data for Efficient Storage:
Advanced Spark SQL Techniques
- 📊 Using Temporary Views for SQL Queries:
df.createOrReplaceTempView('temp_view') spark.sql('SELECT * FROM temp_view WHERE col > value')
- 🔄 Complex SQL Queries for Data Transformation: Utilizing advanced SQL syntax for complex data transformations.
- 📊 Using Temporary Views for SQL Queries:
Machine Learning Pipelines
- 🤖 Creating and Tuning ML Pipelines: Using PySpark’s MLlib for building and tuning machine learning pipelines.
- 🔧 Feature Engineering in ML Pipelines: Implementing feature transformers and selectors within ML pipelines.
Integration with Other Big Data Tools
- 📂 Reading and Writing Data to HDFS: Accessing Hadoop Distributed File System (HDFS) for data storage and retrieval.
- 🔄 Interfacing with Kafka for Real-Time Data Processing: Connecting to Apache Kafka for stream processing tasks.
Cloud-Specific PySpark Operations
- ☁️ Utilizing Cloud-Specific Storage Options: Leveraging AWS S3, Azure Blob Storage, or GCP Storage in PySpark.
- 🛠️ Cloud-Based Data Processing Services Integration: Using services like AWS Glue or Azure Synapse for ETL processes.
Security and Compliance in ETL
- 🔐 Implementing Data Encryption and Security: Securing data at rest and in transit during ETL processes.
- 📝 Compliance with Data Protection Regulations: Adhering to GDPR, HIPAA, or other regulations in data processing.
Optimizing ETL Processes for Scalability
- 📈 Dynamic Resource Allocation for ETL Jobs: Adjusting Spark configurations for optimal resource usage.
- 🚀 Best Practices for Scaling ETL Processes: Techniques for scaling ETL pipelines to handle growing data volumes.
If you want to learn more about machines and ETL, please visit my article. If you’re interested in learning about AI, please visit the official website for more information.
39 Comments
Extraproxies.com · 2 August 2024 at 18:41
I’m really enjoying the design and layout of your site. It’s a very easy on the eyes which makes it much more enjoyable for me to come here and visit more often. Did you hire out a designer to create your theme? Superb work!
Arslan Ali · 13 October 2024 at 22:41
Thanks a ton! 😊 I used a WordPress theme as the base and then gave it my own spin. No fancy designer—just a little DIY action! Glad it’s easy on the eyes… saves you from squinting, right? 😄
ProxiesLive · 21 August 2024 at 02:52
I really like your blog.. very nice colors & theme. Did you create this website yourself or did you hire someone to do it for you? Plz reply as I’m looking to create my own blog and would like to find out where u got this from. thanks a lot
Arslan Ali · 13 October 2024 at 22:41
Thank you so much! 😊 I used a WordPress theme and customized it myself—didn’t hire anyone. If you’re starting a blog, WordPress has a lot of great options to get you going. Happy to help if you need any tips!
Jerry Gemmell · 28 August 2024 at 22:54
Thank you so much for giving everyone an extremely breathtaking possiblity to check tips from this website. It’s usually very enjoyable plus jam-packed with a lot of fun for me and my office friends to search your web site particularly thrice in 7 days to see the newest guides you have got. Of course, I’m certainly motivated concerning the breathtaking hints you give. Certain 1 points in this post are in reality the most suitable we have all ever had.
Arslan Ali · 13 October 2024 at 22:43
Thanks so much, Jerry! 😊 I’m really glad you’re enjoying the content—it means a lot! Sorry for the delay in my response. How’s everything going with your work or projects lately?
Farhan bagali · 4 September 2024 at 17:16
Assalam walaikum bhai , can you guide me to become Data engineer ( from India )
Arslan Ali · 13 October 2024 at 22:44
Wa alaikum assalam! Sure, bhai! I’ve actually written an article that covers everything you need to know about becoming a data engineer. You can check it out here: Big Data Engineer Career Roadmap( https://codetechguru.com/2024-big-data-engineer-career-roadmap-to-success/ ). Hope it helps! 😊
Arslan Ali · 13 October 2024 at 22:45
Wa alaikum assalam! Sure, bhai! I’ve actually written an article that covers everything you need to know about becoming a data engineer. You can check it out here: Big Data Engineer Career Roadmap ( https://codetechguru.com/2024-big-data-engineer-career-roadmap-to-success/ ). Hope it helps! 😊
Buy Private Proxies · 24 September 2024 at 16:50
This really answered my downside, thank you!
Arslan Ali · 13 October 2024 at 22:46
Glad it helped! 😊 If you have any more questions, feel free to ask!
Dreamproxies · 25 September 2024 at 01:44
as soon as I discovered this site I went on reddit to share some of the love with them.
Arslan Ali · 13 October 2024 at 22:47
Thanks a lot for sharing the love on Reddit! I really appreciate it! 😊
Dreamproxies · 25 September 2024 at 16:44
Some truly nice stuff on this web site, I love it.
Arslan Ali · 13 October 2024 at 22:48
Thank you! So glad you’re enjoying the content! 🙌
Dreamproxies · 26 September 2024 at 18:46
as I website owner I think the subject matter here is really wonderful, appreciate it for your efforts.
Arslan Ali · 13 October 2024 at 22:49
Really appreciate the kind words! It means a lot that you find the content valuable. 😊
Dreamproxies · 26 September 2024 at 20:05
I found your blog site on google and check a number of of your early posts. Continue to keep up the very good operate. I just further up your RSS feed to my MSN News Reader. In search of forward to studying extra from you afterward!…
Arslan Ali · 13 October 2024 at 22:49
Thank you for following the blog and adding it to your RSS feed! I’ll definitely keep up the good work. Looking forward to sharing more with you soon! 🙏
Shared Proxies · 14 October 2024 at 09:39
some truly choice blog posts on this website , saved to favorites.
Private Proxies Top · 23 October 2024 at 00:46
Thank you for the good writeup. It in fact was a amusement account it. Look advanced to far added agreeable from you! By the way, how could we communicate?
PrivateProxies.top · 23 October 2024 at 03:15
Hiya very cool blog!! Guy .. Excellent .. Superb .. I will bookmark your site and take the feeds also…I’m glad to seek out a lot of helpful info here within the post, we want develop more techniques on this regard, thanks for sharing. . . . . .
private proxy · 23 October 2024 at 03:47
fantastic put up, very informative. I’m wondering why the other experts of this sector do not notice this. You should proceed your writing. I am confident, you’ve a huge readers’ base already!
Private Proxies Cheap · 23 October 2024 at 04:52
I just like the valuable info you supply in your articles. I will bookmark your weblog and take a look at again right here regularly. I am somewhat sure I will be told lots of new stuff proper here! Best of luck for the following!
private proxies coupon · 23 October 2024 at 05:26
Thank you, I’ve recently been looking for information about this subject for ages and yours is the best I’ve discovered till now. But, what about the conclusion? Are you sure about the source?
Private Proxies · 23 October 2024 at 06:14
I have acquired some new things from your site about personal computers. Another thing I’ve always believed is that laptop computers have become a product that each house must have for many people reasons. They provide convenient ways in which to organize households, pay bills, shop, study, tune in to music and in many cases watch tv shows. An innovative solution to complete all of these tasks has been a mobile computer. These computer systems are mobile ones, small, highly effective and transportable.
PrivateProxies.top · 23 October 2024 at 08:50
It is best to take part in a contest for one of the best blogs on the web. I will advocate this web site!
private proxies · 23 October 2024 at 11:06
Its like you learn my mind! You appear to know a lot approximately this, such as you wrote the ebook in it or something. I feel that you could do with a few percent to drive the message home a bit, but instead of that, that is magnificent blog. A great read. I will definitely be back.
private proxy · 23 October 2024 at 11:15
Thanks for your marvelous posting! I seriously enjoyed reading it, you might be a great author.I will be sure to bookmark your blog and may come back later on. I want to encourage you to ultimately continue your great posts, have a nice morning!
Private proxy · 23 October 2024 at 12:33
What’s Happening i am new to this, I stumbled upon this I have found It absolutely helpful and it has aided me out loads. I hope to contribute & aid other users like its aided me. Good job.
Private Proxies Cheap · 23 October 2024 at 12:37
I have discovered that costs for on-line degree authorities tend to be a terrific value. For instance a full 4-year college Degree in Communication in the University of Phoenix Online consists of 60 credits with $515/credit or $30,900. Also American Intercontinental University Online gives a Bachelors of Business Administration with a overall program requirement of 180 units and a cost of $30,560. Online learning has made obtaining your college degree so much easier because you may earn your degree from the comfort of your home and when you finish from work. Thanks for other tips I have learned from your blog.
Private Proxies Coupon · 23 October 2024 at 16:12
Greetings from Ohio! I’m bored at work so I decided to check out your site on my iphone during lunch break. I really like the info you present here and can’t wait to take a look when I get home. I’m surprised at how quick your blog loaded on my mobile .. I’m not even using WIFI, just 3G .. Anyhow, good blog!
Proxies Private · 23 October 2024 at 17:02
As a Newbie, I am continuously browsing online for articles that can be of assistance to me. Thank you
privateproxies · 23 October 2024 at 17:59
Someone essentially assist to make critically posts I might state. This is the very first time I frequented your web page and thus far? I amazed with the analysis you made to create this particular submit incredible. Wonderful activity!
PrivateProxies.top · 23 October 2024 at 19:15
It’s the best time to make some plans for the future and it is time to be happy. I have read this post and if I could I wish to suggest you some interesting things or advice. Perhaps you could write next articles referring to this article. I wish to read more things about it!
PrivateProxies.top · 23 October 2024 at 21:35
Hey! Someone in my Myspace group shared this website with us so I came to take a look. I’m definitely loving the information. I’m book-marking and will be tweeting this to my followers! Excellent blog and great design.
Private proxies buy · 23 October 2024 at 21:49
I’ll right away grab your rss feed as I can’t find your email subscription link or e-newsletter service. Do you have any? Please let me know so that I could subscribe. Thanks.
10000 Cheap Proxies · 3 December 2024 at 13:29
I went over this web site and I believe you have a lot of great information, saved to fav (:.
Buy 10000 Proxies · 8 December 2024 at 10:26
Only a smiling visitor here to share the love (:, btw outstanding design and style.